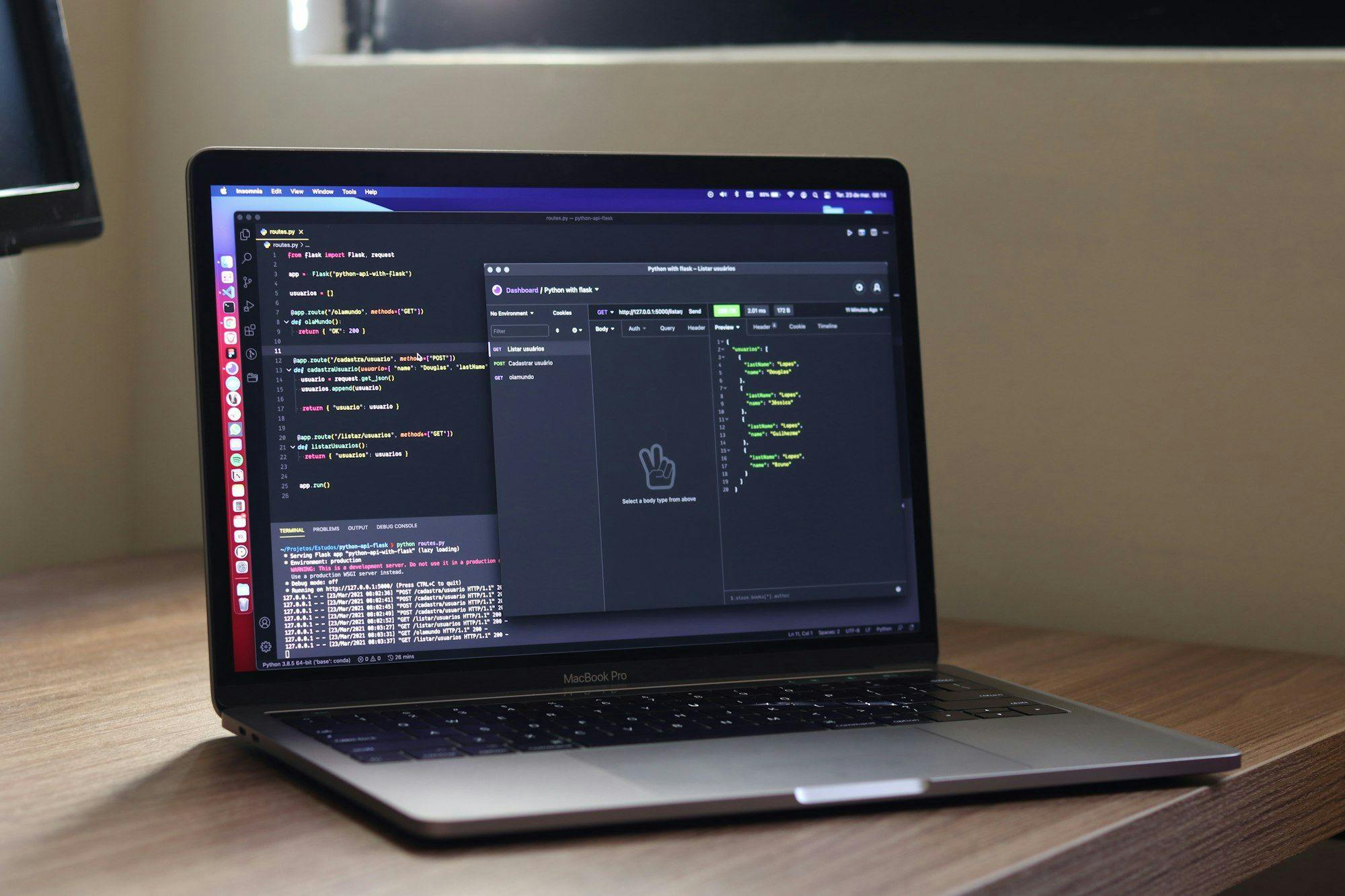
Fetching metadata and content from IPFS (InterPlanetary File System) using the Indexer API involves a few steps, given that IPFS is a protocol and peer-to-peer network for storing and sharing data in a distributed file system. The Indexer API, depending on the context, typically refers to a service that indexes blockchain data, including links to IPFS where NFT (Non-Fungible Token) metadata and files are often stored.
Here’s a general approach on how to fetch metadata and IPFS content using an Indexer API, with a focus on NFTs as a common use case:
Step 1: Choose an Indexer API
First, select an appropriate Indexer API that supports the blockchain network of your NFTs. Services like The Graph, Alchemy, Moralis, or specific blockchain explorers offer APIs that can index and query blockchain data, including NFT metadata.
Factors to Consider When Choosing an Indexer API:
- Blockchain Compatibility: Ensure the API supports your NFT's blockchain.
- Data Freshness: Look for low-latency, up-to-date data retrieval.
- Query Flexibility: Choose APIs that offer robust search and filter tools.
- Scalability and Reliability: The API should handle high request volumes reliably.
- Ease of Use: Favor APIs with clear documentation and developer support.
- Cost: Consider the pricing structure and choose one that fits your budget.
The best Indexer API depends on your project's blockchain network, data query complexity, scalability needs, and budget. Experiment with several in a development environment to identify the best fit for your application.
Step 2: Query the Indexer API for NFT Metadata
Using the Indexer API, you can query for NFT metadata, which often includes IPFS links. These queries require knowing the smart contract address of the NFT collection and the token ID of the specific NFT you're interested in. The Indexer API can return various pieces of information, including a URL pointing to the NFT's metadata on IPFS.
Query Construction
Format Your Query: Depending on the chosen Indexer API's format (REST or GraphQL), construct your query by including the necessary parameters (contract address and token ID).
1. Query Construction for REST APIs
REST APIs are based on standard web protocols (HTTP), and interacting with them involves crafting a URL that includes your request parameters.
- Understand the Endpoint: Each REST API has specific endpoints (URLs) for different types of data. First, find the endpoint URL that is designed to retrieve NFT metadata.
- Include Parameters in the URL: Add the smart contract address and token ID to your request. These are usually appended to the URL as query parameters.
- Use the GET Method: For retrieving data, the GET method is typically used. This means you're essentially asking the server to send data back to you based on the parameters in your URL.
2. Query Construction for GraphQL APIs
GraphQL APIs allow for more tailored requests, letting you specify exactly what data you want back, which can help reduce bandwidth and speed up response times.
- Define Your Query: Start by writing a query that tells the GraphQL server what data you're looking for. You'll use a specific syntax to define the query structure.
- Specify Parameters: In your query, include variables for the smart contract address and token ID. This might look like:
Retrieval and Extraction of Metadata URL
Parsing the Response:
Once you receive the response, you'll need to parse the JSON to find the metadata URL or IPFS link. This involves navigating through the JSON structure, which may vary depending on the API. Look for fields like metadata, image, or external_url.
- Extracting IPFS Links: If the metadata URL is an IPFS link, it will likely start with ipfs://. To access the file on the web, you'll need to convert this link to an HTTP URL by prefixing it with an IPFS gateway URL, such as https://ipfs.io/ipfs/.
- Accessing the Metadata: Use the extracted URL or converted IPFS link in a web browser or another HTTP request to access the actual metadata file. This file is usually in JSON format and contains detailed information about the NFT, such as its name, description, and image.
Metadata Utilization
Access and Parse Metadata: Use an IPFS gateway to convert the IPFS link to a web-accessible URL, then fetch and parse the metadata. This JSON file typically includes details such as the NFT's name, description, image, and other attributes.
Optimization and Handling
Optimize with Caching
- Purpose: Caching saves copies of frequently accessed data, reducing the need to repeatedly fetch data from the source, which can be slow and resource-intensive.
- Implementation: You can implement caching by storing the retrieved NFT metadata in a local database, in-memory store (like Redis), or even the user's browser (using local storage for web applications). When a request for NFT metadata is made, your system should first check if a cached version exists and is up-to-date before deciding to fetch it from the IPFS or the API.
- Benefits: This reduces load times for your users and decreases the number of API calls you make, which is especially important for services with rate limits or costs per request.
Rate Limit Management
APIs often limit the number of requests a user can make in a certain timeframe to prevent abuse and overload. Exceeding these limits can result in your requests being blocked.
Strategies:
1- Throttling: Implement request throttling in your application to spread out API calls over time, ensuring you stay within the rate limit.
2- Backoff Algorithms: Implement exponential backoff algorithms for retrying failed requests, gradually increasing the delay between retries to reduce the likelihood of hitting the rate limit.
3- Monitoring: Actively monitor your API usage to adjust your request patterns as needed and to stay informed about your current rate limit consumption.
Robust Error Handling
Network issues, API changes, or unavailable metadata can cause errors in your application. Anticipating and planning for these errors is crucial for maintaining a good user experience.
Implementation:
1- Try-Catch Blocks: Use try-catch blocks in your code to gracefully handle exceptions that occur during API calls or data processing.
2- User Feedback: Provide clear, user-friendly error messages or fallback content if metadata cannot be retrieved. This ensures users are not left with blank screens or cryptic error codes.
3- Automated Alerts: Implement logging and alerting mechanisms to notify you of errors, especially those that occur frequently or indicate larger issues with your metadata retrieval process.
Example Query (Pseudocode):
Javascript:
Step 3: Fetch NFT Metadata from IPFS
Once you have the IPFS URL from the metadata, you can fetch the metadata directly from IPFS. If the URL is an IPFS link (usually starting with ipfs://), you might need to convert it to an HTTP gateway URL. This is because browsers cannot directly resolve ipfs:// links without a compatible extension or gateway.
- Start with the IPFS Link: Your starting point is the IPFS link found in the NFT metadata, which typically begins with ipfs:// followed by a unique content identifier (CID).
- Convert the IPFS Link to HTTP: To make the IPFS link web-accessible, you convert it to an HTTP URL by using an IPFS gateway. An IPFS gateway serves as a bridge between the IPFS network and the traditional HTTP web. You replace the ipfs:// part of the link with the URL of a gateway, such as https://ipfs.io/ipfs/. This results in a new URL that looks something like https://ipfs.io/ipfs/<CID>.
- Fetch the Metadata: With the converted HTTP URL, you can now fetch the metadata using any standard web browser or through a programming request. If you’re coding, you might use JavaScript's fetch API or Python's requests library to make an HTTP request to the converted URL. This request retrieves the metadata file from IPFS through the gateway.
Convert IPFS URL to HTTP:
Javascript:
Step 4: Accessing Content within Metadata
NFT metadata typically includes links to the actual content (e.g., images, videos) stored on IPFS. You would follow a similar process to convert any IPFS links within the metadata to HTTP URLs to access the content.
- Retrieve and Parse Metadata: Once you've fetched the NFT's metadata from IPFS (as described in previous steps), the next step is to parse this JSON file to find the content links. Look for fields such as image, animation_url, or other custom fields that might contain IPFS URLs to the NFT's associated digital assets.
- Convert IPFS Links to HTTP URLs: Similar to accessing the metadata itself, you'll need to convert any IPFS links found within the metadata to HTTP URLs. This conversion allows these assets to be accessed through standard web browsers and applications. Use an IPFS gateway by replacing the ipfs:// part of the link with the gateway's base URL, like https://ipfs.io/ipfs/.
- Access the Digital Content: With the converted HTTP URLs, you can now access the NFT's digital assets directly. This might involve displaying an image or video in a web application, downloading the content for personal use, or further processing the data as required by your application.
Considerations
- IPFS Gateway: While ipfs.io is a commonly used public gateway, there are many others, and you can even run your own IPFS node for direct access to the network.
- API Rate Limits and Keys: Be mindful of any rate limits imposed by the Indexer API or IPFS gateways. Some services may require API keys for access.
- Data Structure: The structure of the response from the Indexer API and the format of the NFT metadata can vary. Always refer to the specific documentation for the API you're using.
Conclusion
Learning to fetch metadata and content from IPFS using an Indexer API is essential. This skill facilitates the integration of digital assets, like NFTs, into applications, combining blockchain's secure data handling with IPFS's decentralized storage. It requires mastering API use, converting IPFS links for web access, and understanding data management principles. Mastering this enables the creation of innovative, accessible digital experiences within the decentralized ecosystem.
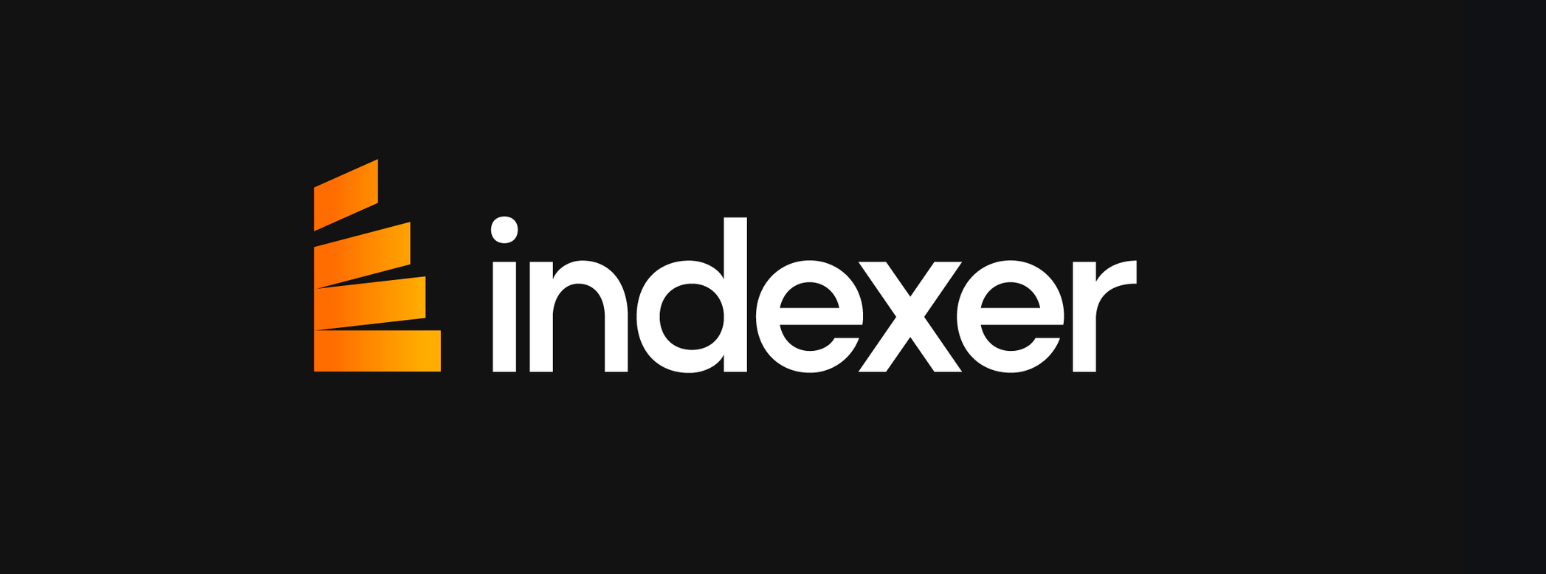
Curious about elevating your app with the power of blockchain! Harness the Indexer API for easy metadata and IPFS content integration at Indexer.xyz. Simplify your app's access to digital assets across various blockchains, enhancing user experience and functionality. Visit Indexer.xyz now and lead your application into the future with the ultimate multichain capabilities. Visit today!